手势事件
GestureDetector
GestureDetector
是一个用于手势识别的功能性组件,我们通过它可以来识别各种手势。
定义
GestureDetector({
Key? key,
this.child,
// 单击
this.onTapDown,
this.onTapUp,
this.onTap,
this.onTapCancel,
// 双指
this.onSecondaryTap,
this.onSecondaryTapDown,
this.onSecondaryTapUp,
this.onSecondaryTapCancel,
// 三点
this.onTertiaryTapDown,
this.onTertiaryTapUp,
this.onTertiaryTapCancel,
// 双击
this.onDoubleTapDown,
this.onDoubleTap,
this.onDoubleTapCancel,
// 长按
this.onLongPressDown,
this.onLongPressCancel,
this.onLongPress,
this.onLongPressStart,
this.onLongPressMoveUpdate,
this.onLongPressUp,
this.onLongPressEnd,
// 两点 三点 长按
this.onSecondaryLongPressDown,
this.onSecondaryLongPressCancel,
this.onSecondaryLongPress,
this.onSecondaryLongPressStart,
this.onSecondaryLongPressMoveUpdate,
this.onSecondaryLongPressUp,
this.onSecondaryLongPressEnd,
this.onTertiaryLongPressDown,
this.onTertiaryLongPressCancel,
this.onTertiaryLongPress,
this.onTertiaryLongPressStart,
this.onTertiaryLongPressMoveUpdate,
this.onTertiaryLongPressUp,
this.onTertiaryLongPressEnd,
// 垂直 水平 Drag
this.onVerticalDragDown,
this.onVerticalDragStart,
this.onVerticalDragUpdate,
this.onVerticalDragEnd,
this.onVerticalDragCancel,
this.onHorizontalDragDown,
this.onHorizontalDragStart,
this.onHorizontalDragUpdate,
this.onHorizontalDragEnd,
this.onHorizontalDragCancel,
this.onForcePressStart,
this.onForcePressPeak,
this.onForcePressUpdate,
this.onForcePressEnd,
// 点击滑动
this.onPanDown,
this.onPanStart,
this.onPanUpdate,
this.onPanEnd,
this.onPanCancel,
this.onScaleStart,
this.onScaleUpdate,
this.onScaleEnd,
this.behavior,
this.excludeFromSemantics = false,
this.dragStartBehavior = DragStartBehavior.start,
包含了大量的交互操作
代码
import 'package:flutter/material.dart';
class GesturePage extends StatefulWidget {
const GesturePage({Key? key}) : super(key: key);
State<GesturePage> createState() => _GesturePageState();
}
class _GesturePageState extends State<GesturePage> {
double? dx, dy;
Widget _buildView() {
return GestureDetector(
child: Container(
color: Colors.amber,
width: 200,
height: 200,
),
// 点击
onTap: () {
print('点击 onTap');
},
// 长按
onLongPress: () {
print('长按 onLongPress');
},
// 双击
onDoubleTap: () {
print('双击 onLongPress');
},
// 按下
onPanDown: (DragDownDetails e) {
print("按下 ${e.globalPosition}");
},
// 按下滑动
onPanUpdate: (DragUpdateDetails e) {
setState(() {
dx = e.delta.dx;
dy = e.delta.dy;
});
},
// 松开
onPanEnd: (DragEndDetails e) {
print(e.velocity);
},
);
}
Widget build(BuildContext context) {
return Scaffold(
body: SizedBox.expand(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
_buildView(),
// 显示
Text('x: $dx, y: $dy'),
],
),
),
);
}
}
输出
flutter: 用户手指按下:Offset(127.7, 218.0)
flutter: Velocity(0.0, 0.0)
Reloaded 1 of 585 libraries in 171ms.
Reloaded 2 of 585 libraries in 205ms.
flutter: 按下 Offset(128.0, 188.2)
flutter: 长按 onLongPress
flutter: 按下 Offset(145.0, 214.2)
flutter: Velocity(-44.4, -45.0)
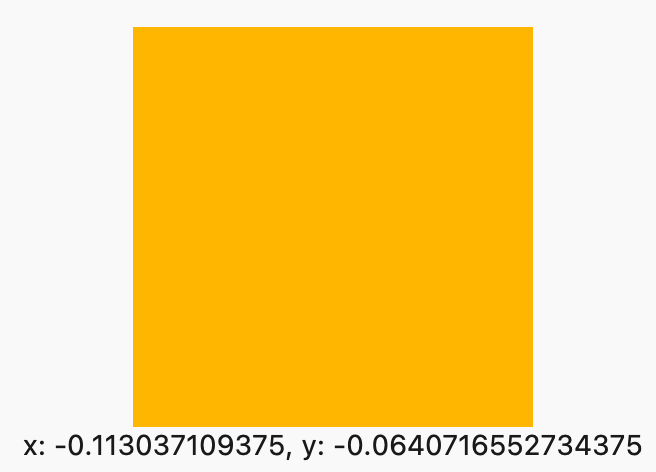
InkWell
InkWell 是带有水波纹的点击事件的组件
定义
const InkWell({
Key? key,
Widget? child,
// 点击
GestureTapCallback? onTap,
GestureTapCallback? onDoubleTap,
// 长按
GestureLongPressCallback? onLongPress,
GestureTapDownCallback? onTapDown,
GestureTapCancelCallback? onTapCancel,
ValueChanged<bool>? onHighlightChanged,
ValueChanged<bool>? onHover,
// 光标样式
MouseCursor? mouseCursor,
// 颜色
Color? focusColor,
Color? hoverColor,
Color? highlightColor,
MaterialStateProperty<Color?>? overlayColor,
Color? splashColor,
InteractiveInkFeatureFactory? splashFactory,
double? radius,
BorderRadius? borderRadius,
ShapeBorder? customBorder,
bool? enableFeedback = true,
bool excludeFromSemantics = false,
FocusNode? focusNode,
bool canRequestFocus = true,
ValueChanged<bool>? onFocusChange,
bool autofocus = false,
})
代码
import 'package:flutter/material.dart';
class InkWellPage extends StatelessWidget {
const InkWellPage({Key? key}) : super(key: key);
Widget _buildView() {
return InkWell(
// 点击
onTap: () {},
// 水波纹颜色
splashColor: Colors.blue,
// 高亮颜色
highlightColor: Colors.yellow,
// 鼠标滑过颜色
hoverColor: Colors.brown,
//
child: const Text(
'点我 InkWell',
style: TextStyle(
fontSize: 50,
),
),
);
}
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: _buildView(),
),
);
}
}
输出
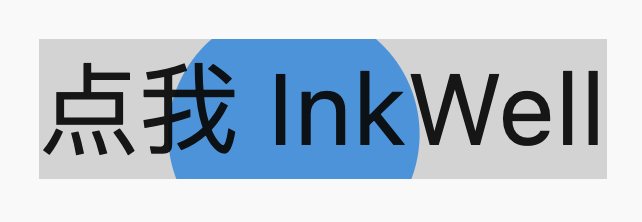
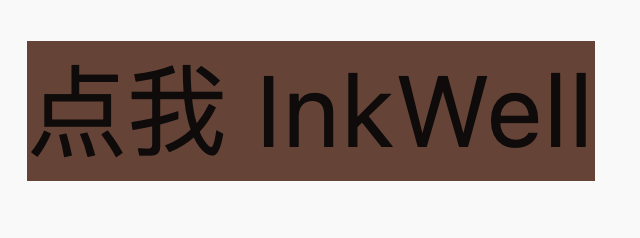
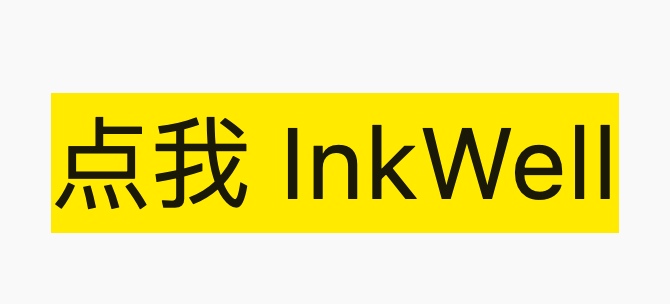