样式管理 Theme
ThemeData 样式对象
- 定义
factory ThemeData({
// 全局配置
AndroidOverscrollIndicator? androidOverscrollIndicator,
bool? applyElevationOverlayColor,
NoDefaultCupertinoThemeData? cupertinoOverrideTheme,
InputDecorationTheme? inputDecorationTheme,
MaterialTapTargetSize? materialTapTargetSize,
PageTransitionsTheme? pageTransitionsTheme,
TargetPlatform? platform,
ScrollbarThemeData? scrollbarTheme,
InteractiveInkFeatureFactory? splashFactory,
VisualDensity? visualDensity,
bool? useMaterial3,
// 颜色管理
ColorScheme? colorScheme,
Color? colorSchemeSeed,
Brightness? brightness,
MaterialColor? primarySwatch,
Color? primaryColor,
Color? primaryColorLight,
Color? primaryColorDark,
Color? focusColor,
Color? hoverColor,
Color? shadowColor,
Color? canvasColor,
Color? scaffoldBackgroundColor,
Color? bottomAppBarColor,
Color? cardColor,
Color? dividerColor,
Color? highlightColor,
Color? splashColor,
Color? selectedRowColor,
Color? unselectedWidgetColor,
Color? disabledColor,
Color? secondaryHeaderColor,
Color? backgroundColor,
Color? dialogBackgroundColor,
Color? indicatorColor,
Color? hintColor,
Color? errorColor,
Color? toggleableActiveColor,
// 字体和图标
String? fontFamily,
Typography? typography,
TextTheme? textTheme,
TextTheme? primaryTextTheme,
IconThemeData? iconTheme,
IconThemeData? primaryIconTheme,
// 各种组件样式
AppBarTheme? appBarTheme,
MaterialBannerThemeData? bannerTheme,
BottomAppBarTheme? bottomAppBarTheme,
BottomNavigationBarThemeData? bottomNavigationBarTheme,
BottomSheetThemeData? bottomSheetTheme,
ButtonBarThemeData? buttonBarTheme,
ButtonThemeData? buttonTheme,
CardTheme? cardTheme,
CheckboxThemeData? checkboxTheme,
ChipThemeData? chipTheme,
DataTableThemeData? dataTableTheme,
DialogTheme? dialogTheme,
DividerThemeData? dividerTheme,
DrawerThemeData? drawerTheme,
ElevatedButtonThemeData? elevatedButtonTheme,
FloatingActionButtonThemeData? floatingActionButtonTheme,
ListTileThemeData? listTileTheme,
NavigationBarThemeData? navigationBarTheme,
NavigationRailThemeData? navigationRailTheme,
OutlinedButtonThemeData? outlinedButtonTheme,
PopupMenuThemeData? popupMenuTheme,
ProgressIndicatorThemeData? progressIndicatorTheme,
RadioThemeData? radioTheme,
SliderThemeData? sliderTheme,
SnackBarThemeData? snackBarTheme,
SwitchThemeData? switchTheme,
TabBarTheme? tabBarTheme,
TextButtonThemeData? textButtonTheme,
TextSelectionThemeData? textSelectionTheme,
TimePickerThemeData? timePickerTheme,
ToggleButtonsThemeData? toggleButtonsTheme,
TooltipThemeData? tooltipTheme,
- 我们在 MaterialApp.theme 中初始
lib/main.dart
import 'package:flutter/material.dart';
import 'package:flutter_application_write_docs/pages/theme.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Quick Start',
// 样式
theme: ThemeData(
// 主题颜色
primarySwatch: Colors.brown,
// appBar颜色
appBarTheme: ThemeData.light().appBarTheme.copyWith(
backgroundColor: Colors.green,
foregroundColor: Colors.white,
),
// 按钮颜色
elevatedButtonTheme: ElevatedButtonThemeData(
style: ElevatedButton.styleFrom(
onPrimary: Colors.white,
primary: Colors.amber,
),
),
),
// page
home: const ThemePage(),
// 关闭 debug 标签
debugShowCheckedModeBanner: false,
);
}
}
lib/pages/theme.dart
import 'package:flutter/material.dart';
class ThemePage extends StatelessWidget {
const ThemePage({Key? key}) : super(key: key);
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('ThemePage'),
),
body: Center(
child: ElevatedButton(
onPressed: () {},
child: const Text('Theme'),
),
),
);
}
}
输出
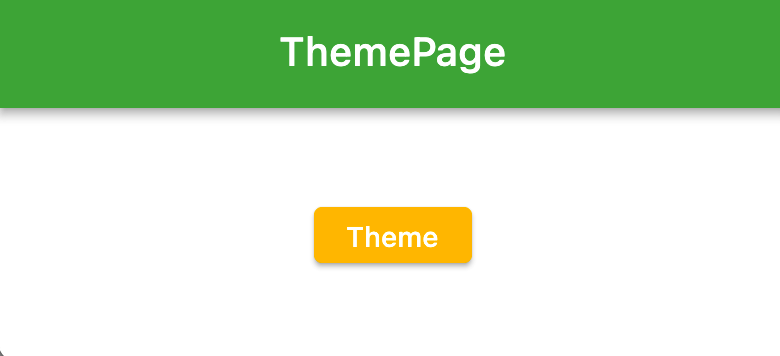
Color 与 MaterialColor
- Color 颜色
https://api.flutter.dev/flutter/material/Colors-class.html
如设计稿的颜色是 #40c254
, 转换成 16 进制颜色
// 字符串转 Color
Color stringToColor(String source) {
return Color(int.parse(source, radix: 16) | 0xFF000000);
}
Color c = stringToColor("40c254");
- MaterialColor 色彩表
https://api.flutter.dev/flutter/material/MaterialColor-class.html
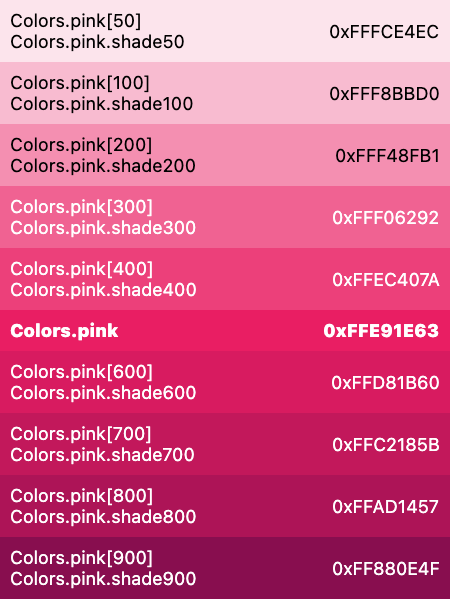
// 字符串转 MaterialColor
MaterialColor stringToMaterialColor(String source) {
Color color = stringToColor(source);
List<double> strengths = <double>[.05];
Map<int, Color> swatch = <int, Color>{};
final int r = color.red, g = color.green, b = color.blue;
for (int i = 1; i < 10; i++) {
strengths.add(0.1 * i);
}
for (var strength in strengths) {
final double ds = 0.5 - strength;
swatch[(strength * 1000).round()] = Color.fromRGBO(
r + ((ds < 0 ? r : (255 - r)) * ds).round(),
g + ((ds < 0 ? g : (255 - g)) * ds).round(),
b + ((ds < 0 ? b : (255 - b)) * ds).round(),
1,
);
}
return MaterialColor(color.value, swatch);
}
MaterialColor mc = stringToColor("40c254");
- 代码
import 'package:flutter/material.dart';
// 字符串转 Color
Color stringToColor(String source) {
return Color(int.parse(source, radix: 16) | 0xFF000000);
}
// 字符串转 MaterialColor
MaterialColor stringToMaterialColor(String source) {
Color color = stringToColor(source);
List<double> strengths = <double>[.05];
Map<int, Color> swatch = <int, Color>{};
final int r = color.red, g = color.green, b = color.blue;
for (int i = 1; i < 10; i++) {
strengths.add(0.1 * i);
}
for (var strength in strengths) {
final double ds = 0.5 - strength;
swatch[(strength * 1000).round()] = Color.fromRGBO(
r + ((ds < 0 ? r : (255 - r)) * ds).round(),
g + ((ds < 0 ? g : (255 - g)) * ds).round(),
b + ((ds < 0 ? b : (255 - b)) * ds).round(),
1,
);
}
return MaterialColor(color.value, swatch);
}
class ColorPage extends StatelessWidget {
const ColorPage({Key? key}) : super(key: key);
Widget build(BuildContext context) {
var c = stringToColor("FFB822");
var mc = stringToMaterialColor("5C78FF");
return Scaffold(
body: SizedBox.expand(
child: Column(
children: [
// Color
Container(
color: c,
height: 50,
),
// MaterialColor
for (var i = 1; i < 10; i++)
Container(
color: mc[i * 100],
height: 50,
),
],
),
),
);
}
}
- 输出
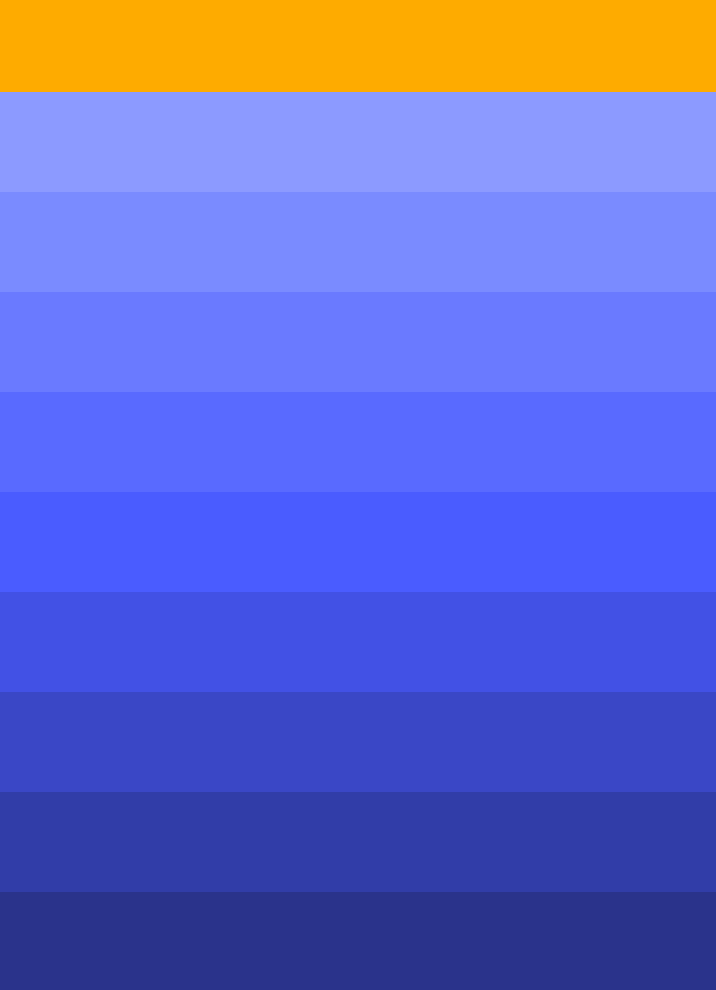