对齐定位 Align
调整子元素在父元素的位置
https://api.flutter.dev/flutter/widgets/Align-class.html
Align
定义
Align({
Key key,
// 需要一个AlignmentGeometry类型的值
// AlignmentGeometry 是一个抽象类,
// 它有两个常用的子类:Alignment和 FractionalOffset
this.alignment = Alignment.center,
// 两个缩放因子
// 会分别乘以子元素的宽、高,最终的结果就是 Align 组件的宽高
this.widthFactor,
this.heightFactor,
Widget child,
})
代码
import 'package:flutter/material.dart';
class AlignPage extends StatelessWidget {
const AlignPage({Key? key}) : super(key: key);
Widget build(BuildContext context) {
return const Scaffold(
body: Align(
widthFactor: 2,
heightFactor: 2,
alignment: Alignment.bottomLeft,
child: FlutterLogo(
size: 50,
),
),
);
}
}
输出
可以发现,Align 的高宽=子元素高宽*高宽因子 factor,如果 null 采用父元素高度约束
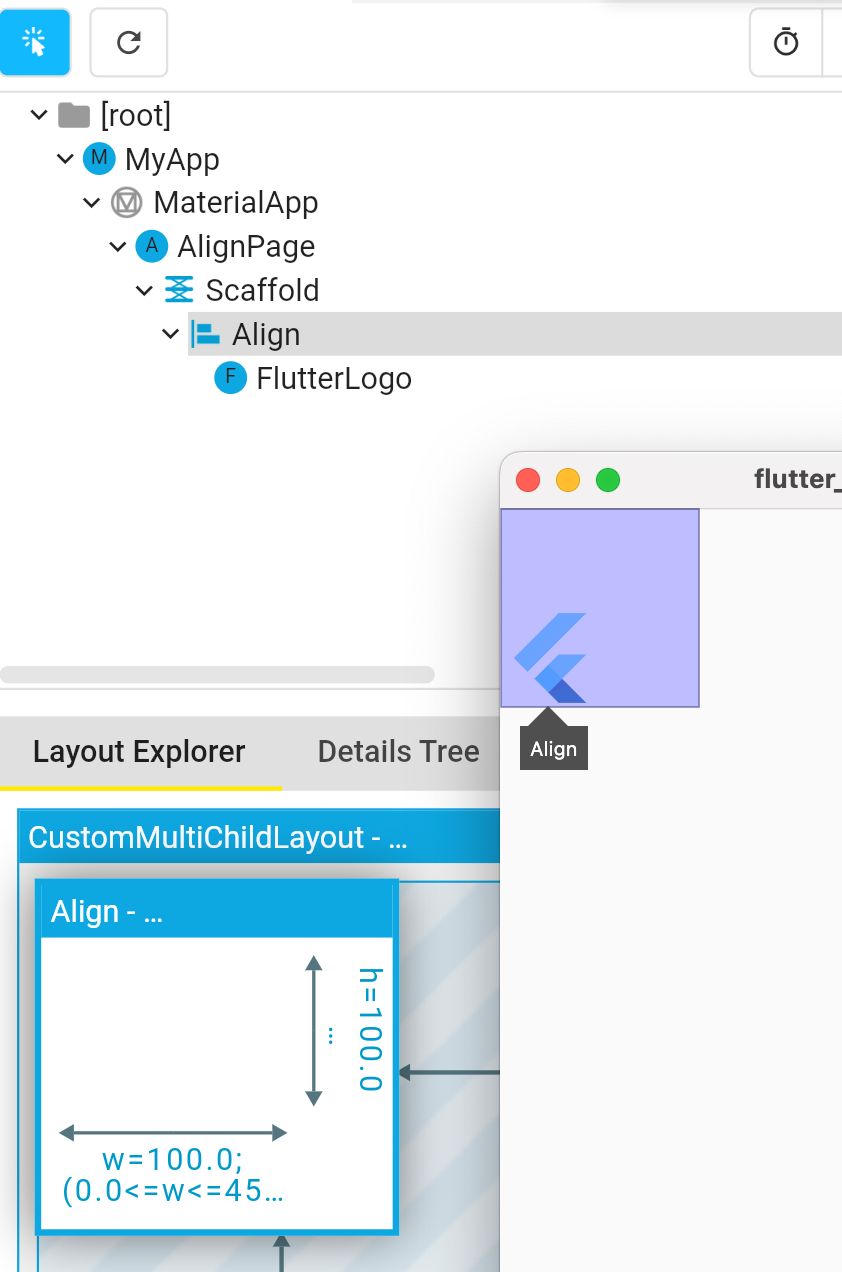
Alignment
Alignment 是从 Align 的中心点出发
定义
/// The top left corner.
static const Alignment topLeft = Alignment(-1.0, -1.0);
/// The center point along the top edge.
static const Alignment topCenter = Alignment(0.0, -1.0);
/// The top right corner.
static const Alignment topRight = Alignment(1.0, -1.0);
/// The center point along the left edge.
static const Alignment centerLeft = Alignment(-1.0, 0.0);
/// The center point, both horizontally and vertically.
static const Alignment center = Alignment(0.0, 0.0);
/// The center point along the right edge.
static const Alignment centerRight = Alignment(1.0, 0.0);
/// The bottom left corner.
static const Alignment bottomLeft = Alignment(-1.0, 1.0);
/// The center point along the bottom edge.
static const Alignment bottomCenter = Alignment(0.0, 1.0);
/// The bottom right corner.
static const Alignment bottomRight = Alignment(1.0, 1.0);
Alignment(-1.0, -1.0) 标识从中心点出发,左上角
代码
...
return const Scaffold(
body: Align(
alignment: Alignment(-1, -1),
child: FlutterLogo(
size: 50,
),
),
);
输出
Alignment.topLeft == Alignment(-1, -1) ,用哪种方式都可以
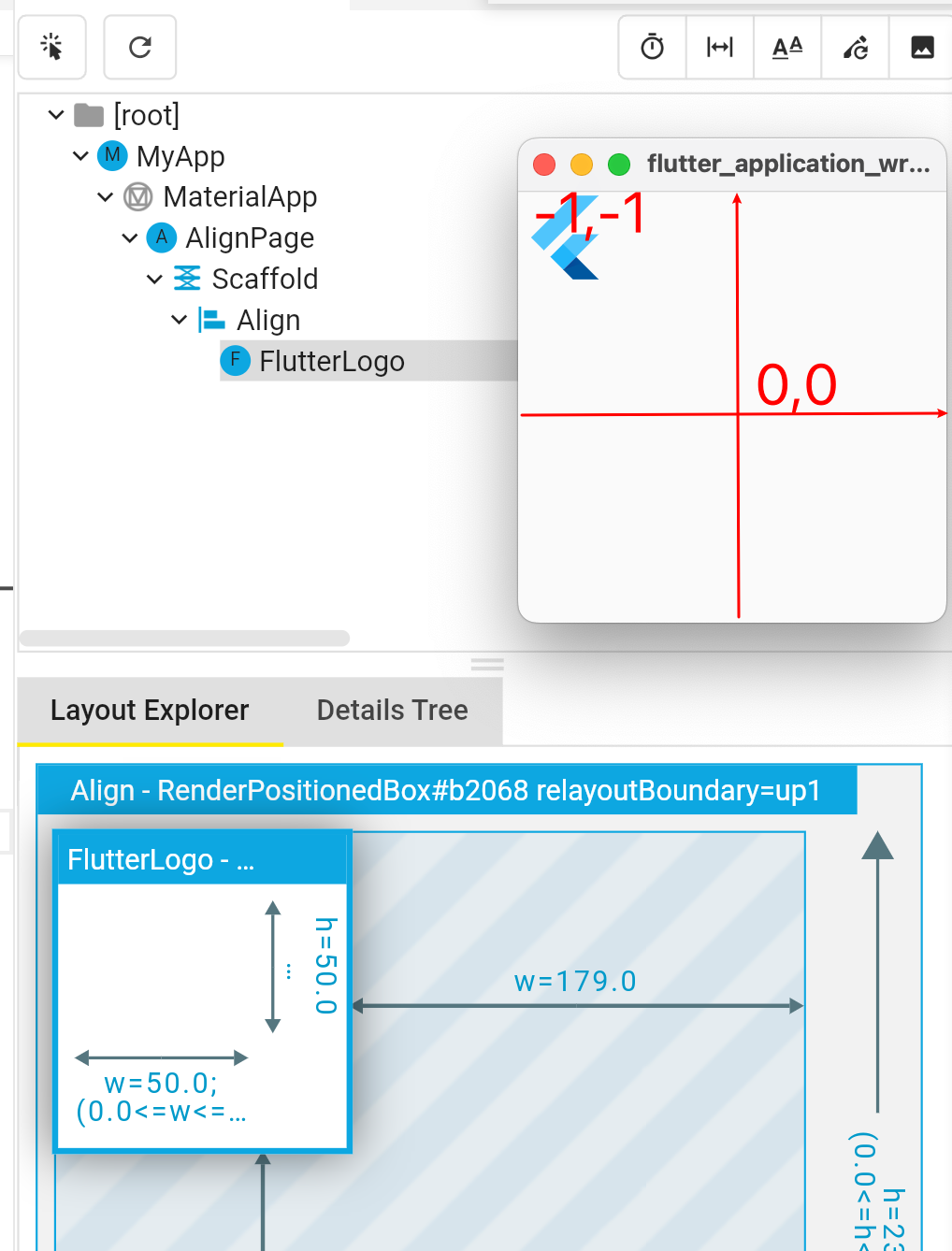
FractionalOffset
这种方式是固定从左上角出发
代码
...
Widget build(BuildContext context) {
return const Scaffold(
body: Align(
alignment: FractionalOffset(0.5, 0.1),
child: FlutterLogo(
size: 50,
),
),
);
}
用 FractionalOffset 对象,输入 0~1 的比例值
输出
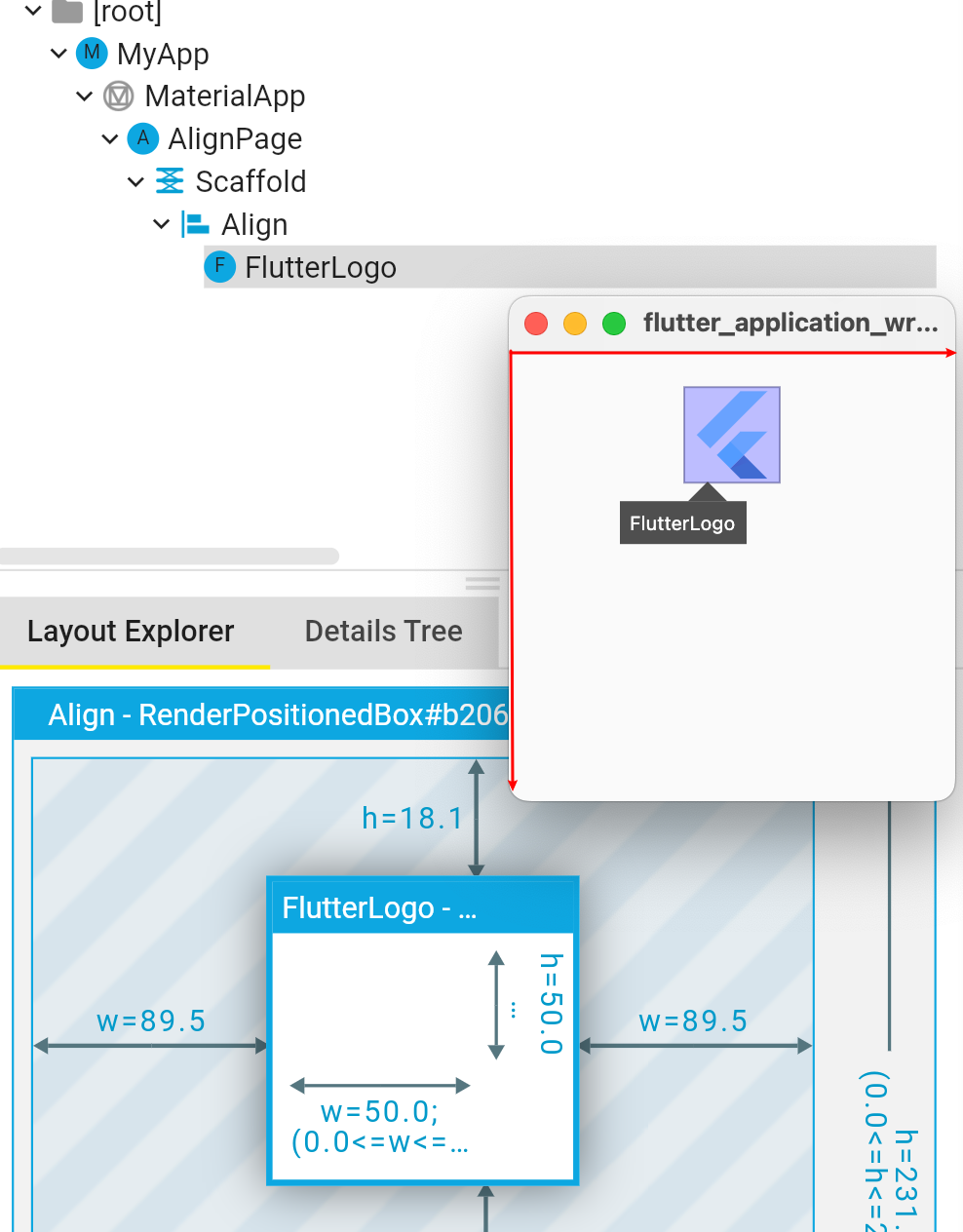
Center
Center 是集成了 Align 对象,默认 alignment=Alignment.center
Center 定义, 少了一个 alignment 参数
class Center extends Align {
/// Creates a widget that centers its child.
const Center({ Key? key, double? widthFactor, double? heightFactor, Widget? child })
: super(key: key, widthFactor: widthFactor, heightFactor: heightFactor, child: child);
}
然后 Align 定义, 默认了 this.alignment = Alignment.center,
class Align extends SingleChildRenderObjectWidget {
/// Creates an alignment widget.
///
/// The alignment defaults to [Alignment.center].
const Align({
Key? key,
this.alignment = Alignment.center,
this.widthFactor,
this.heightFactor,
Widget? child,
}) : assert(alignment != null),
assert(widthFactor == null || widthFactor >= 0.0),
assert(heightFactor == null || heightFactor >= 0.0),
super(key: key, child: child);
代码
...
Widget build(BuildContext context) {
return const Scaffold(
body: Center(
child: FlutterLogo(
size: 50,
),
),
);
}
输出
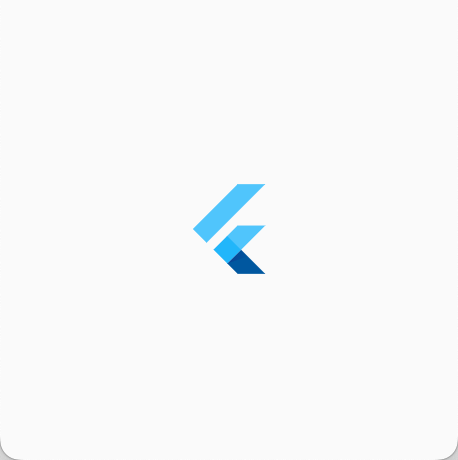